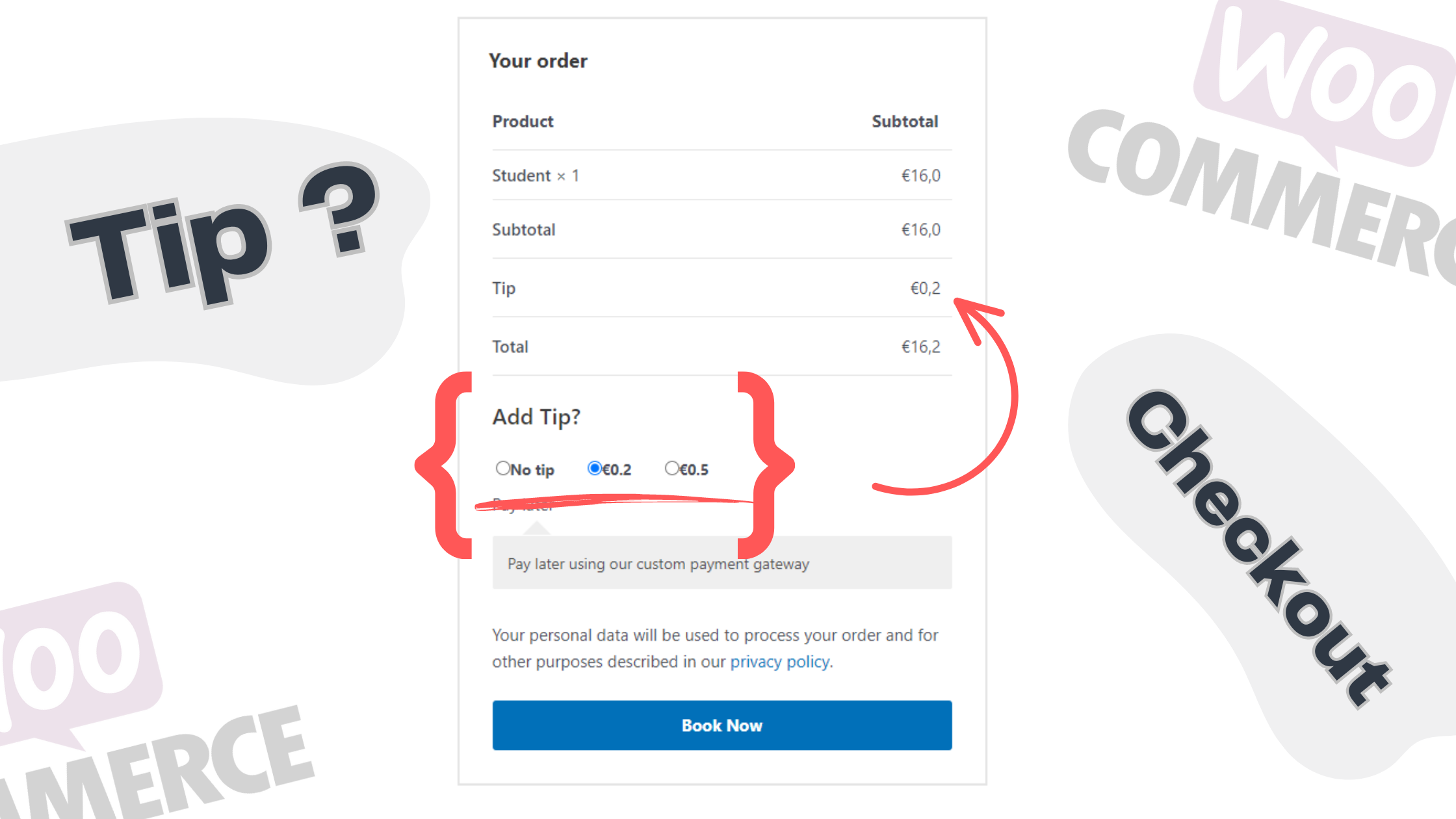
This code is a WooCommerce customization that allows customers to add a tip to their order during checkout.
Advantages of the tip option:
- Increases Revenue: Adding a tip option gives customers an opportunity to show appreciation for great service, and this can result in increased revenue for the business.
- Improves Customer Satisfaction: Allowing customers to show their appreciation through a tip option can enhance their overall satisfaction with the business, leading to customer loyalty and repeat business.
- Easy to Implement: Implementing a tip option on WooCommerce checkout is relatively easy, as this code snippet demonstrates.
- Customizable: The code is customizable and can be edited to include different tip amounts or options.
If youre looking for a simpler option, take a look at this nice plugin
<?php
/**
* WooCommerce Tip option on Checkout
*/
// Add The tip
add_action( 'woocommerce_cart_calculate_fees', 'add_tip_fee', 20, 1 );
function add_tip_fee( $cart ) {
if ( is_admin() && ! defined( 'DOING_AJAX' ) ) {
return;
}
// Get the chosen tip amount from the session
$tip_choice = WC()->session->get( 'tip_chosen' );
// Ensure the value is valid before proceeding
if ( isset( $tip_choice ) && is_numeric( $tip_choice ) ) {
$tip_amount = floatval( $tip_choice ); // Convert to float for safety
if ( $tip_amount > 0 ) {
$cart->add_fee( 'Tip', $tip_amount );
}
}
}
// Add tip to order
add_action( 'woocommerce_checkout_update_order_review', 'save_tip_choice' );
function save_tip_choice( $posted_data ) {
parse_str( $posted_data, $output );
if ( isset( $output['tip_choice'] ) ){
WC()->session->set( 'tip_chosen', $output['tip_choice'] );
}
}
// Modify the tip options to include the tip amount in the value
add_action( 'woocommerce_review_order_before_payment', 'checkout_radio_buttons' );
function checkout_radio_buttons() {
echo '<div id="tips" class="tips">';
echo '<h4>Add Tip?</h4>';
// Custom HTML for the radio buttons with input inside label
echo '<div class="form-row-wide update_totals_on_change tip-options">';
echo '<label><input type="radio" name="tip_choice" value="0" checked> No tip</label>';
echo '<label><input type="radio" name="tip_choice" value="0.2">€0.2</label>';
echo '<label><input type="radio" name="tip_choice" value="0.5">€0.5</label>';
echo '<label><input type="radio" name="tip_choice" value="1">€1</label>';
echo '</div>';
echo '</div>';
}
// css
add_action( 'wp_footer', 'checkout_tip_styling' );
function checkout_tip_styling() { ?>
<style>
.tips label {
display: inline !important;
border-radius: 5px;
padding: 8px 15px;
background-color: #f5f6f;
border: 1px solid #d8dee4;
border-radius: 25px;
cursor: pointer;
transition: all 0.2s ease;
}
.tips label:hover {
background-color: #c9c7ff;
}
.tips label:has(input:checked) {
border-color: rgb(37, 99, 235);
background-color: #c9c7ff;
}
.tips input[type="radio"] {
display: none;
}
.tips {
padding: 10px;
background-color: white;
margin-bottom: 20px;
margin-top: -15px;
}
.form-row-wide.update_totals_on_change.tip-options {
display: flex;
gap: 10px;
flex-wrap: wrap;
}
</style>
<?php }
Was this helpful?
YesNo